About Python – “리스트 컴프리헨션, List Comprehension”
About Python – “리스트 컴프리헨션, List Comprehension”
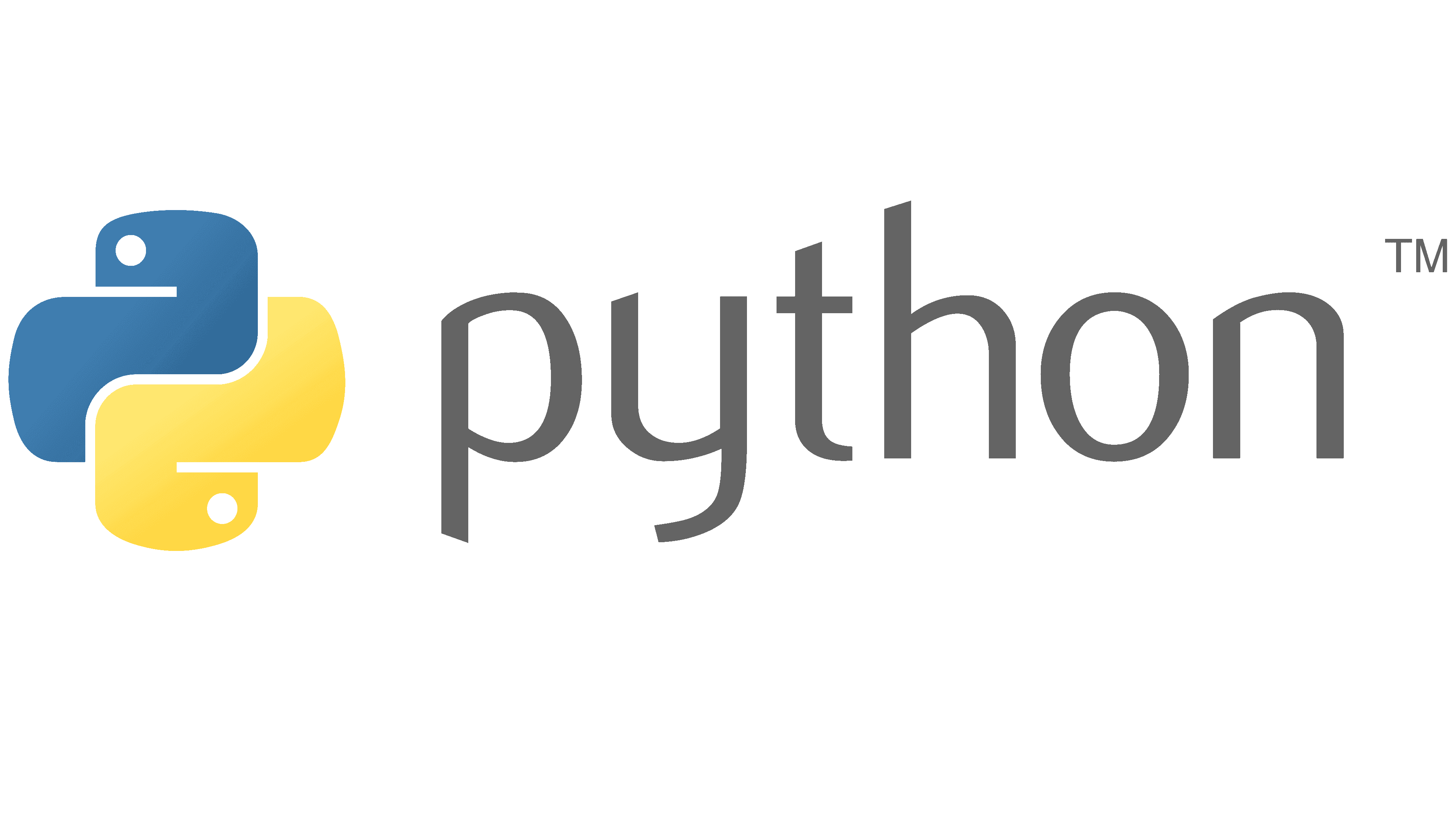
파이썬의 리스트 컴프리헨션 간단한 예제
List comprehension offers a shorter syntax when you want to create a new list based on the values of an existing list.
목록 이해는 기존 목록의 값을 기반으로 새 목록을 만들려는 경우 더 짧은 구문을 제공합니다.
https://www.w3schools.com/python/python_lists_comprehension.asp
animals = ["lion", "monkey", "tiger", "bear", "ant"]
a_animals_list = []
for x in animals:
if "a" in x:
a_animals_list.append(x)
print(a_animals_list)
위와 같은 코드를 살펴봅시다. animals
에 동물들 리스트가 있고, 동물 이름에 a
가 들어간다면 a_animals_list
에 새로운 값을 추가하라는 코드입니다.
이쯤에서 리스트 컴프리헨션을 사용해 볼까요 ?
새 리스트 = [표현식 for item in 반복가능한 객체]
새 리스트 = [표현식 for item in 반복가능한 객체 if 조건 == True]
를 일단 읽어보고 시작해 봅시다.
animals = ["lion", "monkey", "tiger", "bear", "ant"]
a_animals = [x for x in animals if "a" in x]
print(a_animals)
와 같이 나타낼 수 있습니다.
“조건” 부분은 True or False로 판단할 수 있어야 합니다.
animals = ["lion", "monkey", "tiger", "bear", "ant"]
animals_without_lion = [x for x in animals if "lion" != x]
print(animals_without_lion)
전체 리스트에 대해서, x가 “lion” 과 같지 않다면 x를 넣게 됩니다.
animals = ["lion", "monkey", "tiger", "bear", "ant"]
new_animals = [x for x in animals]
print(new_animals)
또한 “조건” 부분은 선택사항이기 때문에 생략이 가능합니다.
list = [f"{n} : 홀수!" for n in range(1,11) if n % 2 == 1]
print(list)
위와 같이 리스트에 들어가는 결과를 입맞에 맞게 설정할 수도 있습니다.
students = {
1 : 'james',
2 : 'smith',
3 : 'nakamura'
}
odd_num_students = {}
for key, value in students.items():
if key % 2 != 0:
odd_num_students[key] = value
print(odd_num_students)
딕셔너리 또한 반복 가능한 객체이므로, 아래와 같이 딕셔너리 컴프리헨션을 사용해서 표현할 수 있습니다.
students = {
1 : 'james',
2 : 'smith',
3 : 'nakamura'
}
odd_num_students = {key: value for key, value in students.items() if key % 2 != 0}
print(odd_num_students)
참고자료 : https://www.w3schools.com/python/python_lists_comprehension.asp